Programmazione Android – Walkmind
CODICE ANDROID
Walkmind è un’applicazione Android sviluppata come prima versione qualche anno fa. Il cuore del software Walkmind è il player dei file audio. Il software deve estrarre il file audio che contiene la domanda e dopo un tempo determinato il corrispondente file audio contenente la risposta. Le opzioni implementate sono già numerose ed esaustive per poter studiare qualsiasi argomento o materia. L’applicazione Walkmind può essere scaricata gratuitamente dal google play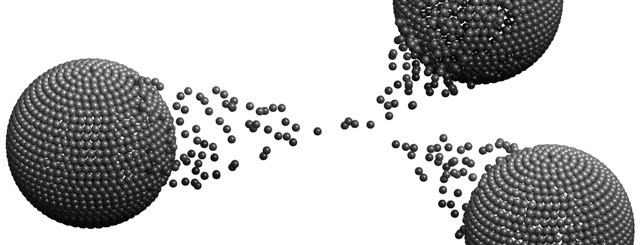
APERTURA DEL PLAYER
Preparazione del Layout. Suddivisione di ogni domanda per argomento o materia. In apertura del Player il codice deve caricare nei vettori stringa e temporali tutti i dati salvati nell’ultima sessione. Ogni domanda e risposta viene abbinata alla materia assegnata. Le azioni scelte per gli oggetti creati nel menù sono implementate nella prima parte del file.// Autore
// Ing. Paolo Sordi, via casilina n. 88 San Cesareo (Roma)
//
package com.idros.walkmind;
import android.app.Activity;
import android.content.Intent;
import android.os.Bundle;
import android.view.View.OnClickListener;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.view.View;
import android.app.AlertDialog;
import android.content.Context;
import java.util.*;
import android.media.MediaPlayer;
import android.media.MediaPlayer.OnCompletionListener;
import java.util.Random;
import java.text.DecimalFormat;
import com.idros.walkmind.R;
import android.os.PowerManager;
import android.content.DialogInterface;
import android.content.DialogInterface.*;
import android.view.KeyEvent;
import android.view.Menu;
import android.view.MenuItem;
import android.view.WindowManager;
import android.content.BroadcastReceiver;
import android.content.IntentFilter;
import android.media.AudioManager;
import android.bluetooth.BluetoothAdapter;
import android.bluetooth.BluetoothDevice;
public class Player_mp3 extends Activity implements OnClickListener{
public Timer timer;
public boolean aggiorna_edit_materia=false;
public short esegui_il_timer_task = 1;
public short indice = -1;
public short intervallo ;
public short num_risposte_date = 0;
public long debug_ritardo = 0;
final short intervallo_salvataggio = 10;
final short intervallo_spegnimento = 30;
short conteggio_allo_spegnimento = 0;
public short num_risposte_al_salvataggio = intervallo_salvataggio;
public int length;
public long durata_wav;
public TextView text_percorso, text_indice, text_durata, text_num_domande, text_save, textView7;
public MyTimerTask myTask;
public String nomefile = "";
public GLOBALCLASS GLOBALE;
public MediaPlayer mp, mp_song , mp_falso;
public Button button1, button2, button9;
public String stringa1, stringa2, stringa3, stringa4, stringa5, stringa6, stringa7, stringa8;
public EditText editText1;
private static PowerManager.WakeLock powerLock;
public void onCreate(Bundle savedInstanceState) {
myTask = null;
MyTimerTask myTask = new MyTimerTask();
GLOBALE = (GLOBALCLASS) getApplicationContext();
super.onCreate(savedInstanceState);
stringa1 = getString(R.string.player_indice);
stringa2 = getString(R.string.player_percentuale);
stringa3 = getString(R.string.n_al_salvataggio);
stringa4 = getString(R.string.STRING_n_domande);
stringa5 = getString(R.string.player_durata);
stringa6 = getString(R.string.player_indice);
stringa7 = getString(R.string.player_file);
stringa8 = getString(R.string.player_time_pausa);
setContentView(R.layout.form_player_mp3);
button2 = (Button) findViewById(R.id.button2);
button2.setOnClickListener(this);
button2.setEnabled(false);
button1 = (Button) findViewById(R.id.button1);
button1.setOnClickListener(this);
final Button button3 = (Button) findViewById(R.id.button3);
button3.setOnClickListener(this);
final Button button4 = (Button) findViewById(R.id.button4);
button4.setOnClickListener(this);
final Button button7 = (Button) findViewById(R.id.button7);
button7.setOnClickListener(this);
final Button button8 = (Button) findViewById(R.id.button8);
button8.setOnClickListener(this);
final Button button9 = (Button) findViewById(R.id.button9);
button9.setOnClickListener(this);
final Button button10 = (Button) findViewById(R.id.button10);
button10.setOnClickListener(this);
text_percorso = (TextView) findViewById(R.id.textView1);
text_percorso.setText(stringa7);
text_indice = (TextView) findViewById(R.id.textView2);
text_indice.setText(stringa6);
text_num_domande = (TextView) findViewById(R.id.textView4);
text_num_domande.setText(stringa4 + GLOBALE.numero_elementi);
textView7 = (TextView) findViewById(R.id.textView7);
textView7.setText(".");
editText1 = (EditText) findViewById(R.id.editText1);
editText1.setText(".");
int i,j;
IntentFilter filter1, filter2, filter3, filter4;
filter1 = new IntentFilter("android.bluetooth.a2dp.profile.action.CONNECTION_STATE_CHANGED");
filter2 = new IntentFilter(android.bluetooth.BluetoothDevice.ACTION_ACL_DISCONNECTED);
filter3 = new IntentFilter(android.bluetooth.BluetoothDevice.ACTION_ACL_CONNECTED);
filter4 = new IntentFilter(android.bluetooth.BluetoothDevice.ACTION_ACL_DISCONNECT_REQUESTED);
this.registerReceiver(mReceiver, filter1);
this.registerReceiver(mReceiver, filter2);
this.registerReceiver(mReceiver, filter3);
this.registerReceiver(mReceiver, filter4);
PowerManager pM = (PowerManager) getSystemService(Context.POWER_SERVICE);
powerLock = pM.newWakeLock(PowerManager.PARTIAL_WAKE_LOCK, "Prevent sleeping");
powerLock.acquire();
boolean non_presente;
GLOBALE.numero_materie =0;
GLOBALE.vettore_elenco_materie_aux = new String[GLOBALE.numero_elementi];
GLOBALE.vettore_elenco_materie_ok_play = new boolean[GLOBALE.numero_elementi];
GLOBALE.vettore_elenco_materie_statistica = new short[GLOBALE.numero_elementi];
// trova tutte le materie presenti
for (i=1; i< GLOBALE.numero_elementi; i++) {
non_presente = true;
if (GLOBALE.vettore_materia[i].equals("null") || GLOBALE.vettore_materia[i] == null || GLOBALE.vettore_materia[i].length()==0) {
GLOBALE.vettore_materia[i] = "*";
}
for (j=0; j< GLOBALE.numero_materie; j++) { if (GLOBALE.vettore_elenco_materie_aux[j].equals(GLOBALE.vettore_materia[i])) { non_presente = false; GLOBALE.vettore_elenco_materie_statistica[j] ++; } } // if (non_presente && !GLOBALE.vettore_materia[i].equals("null") && GLOBALE.vettore_materia[i] != null && GLOBALE.vettore_materia[i].length()>0) {
if (non_presente && GLOBALE.vettore_materia[i].length()>0) {
GLOBALE.vettore_elenco_materie_aux[GLOBALE.numero_materie] = GLOBALE.vettore_materia[i];
GLOBALE.vettore_elenco_materie_statistica[GLOBALE.numero_materie] = 1;
GLOBALE.vettore_elenco_materie_ok_play[GLOBALE.numero_materie] = true;
if (GLOBALE.vettore_materia[i].equals("x1matem")) GLOBALE.vettore_elenco_materie_ok_play[GLOBALE.numero_materie] = false;
if (GLOBALE.vettore_materia[i].equals("x2fisic")) GLOBALE.vettore_elenco_materie_ok_play[GLOBALE.numero_materie] = false;
if (GLOBALE.vettore_materia[i].equals("x3elett")) GLOBALE.vettore_elenco_materie_ok_play[GLOBALE.numero_materie] = false;
if (GLOBALE.vettore_materia[i].equals("x4softw")) GLOBALE.vettore_elenco_materie_ok_play[GLOBALE.numero_materie] = false;
GLOBALE.numero_materie++;
}
}
boolean alfabeto_non_finito = true;
while (alfabeto_non_finito) {
alfabeto_non_finito = false;
for (i=0; i < GLOBALE.numero_materie -1; i++) { int sorteggio = GLOBALE.vettore_elenco_materie_aux[i].toString().compareTo(GLOBALE.vettore_elenco_materie_aux[i+1].toString()); if (sorteggio > 0)
{
short aux = GLOBALE.vettore_elenco_materie_statistica[i+1];
String aux_string = GLOBALE.vettore_elenco_materie_aux[i+1].toString();
boolean aux_boolean = GLOBALE.vettore_elenco_materie_ok_play[i+1];
GLOBALE.vettore_elenco_materie_statistica[i+1] = GLOBALE.vettore_elenco_materie_statistica[i];
GLOBALE.vettore_elenco_materie_aux[i+1] = GLOBALE.vettore_elenco_materie_aux[i];
GLOBALE.vettore_elenco_materie_ok_play[i+1] = GLOBALE.vettore_elenco_materie_ok_play[i];
GLOBALE.vettore_elenco_materie_statistica[i] = aux;
GLOBALE.vettore_elenco_materie_aux[i] = aux_string;
GLOBALE.vettore_elenco_materie_ok_play[i] = aux_boolean;
alfabeto_non_finito = true;
//a is smaller
}
}
}
// Statistica indice di estrazione
GLOBALE.somma_vettore_estrazioni = 0;
for (i=1; i< GLOBALE.numero_elementi; i++) { short indice; indice = GLOBALE.vettore_domande[i]; GLOBALE.somma_vettore_estrazioni += 1/(float)GLOBALE.vettore_estrazione[indice]; } // System.out.println ("%%%%%%%%%%%%%YYYTimerTask-------S " ); Timer timer = new Timer(); if (GLOBALE.numero_elementi > 0) {
intervallo = 1;
timer.schedule(myTask, 1000*intervallo);
intervallo = 15;
}
else
{
GLOBALE.domanda_risposta = -1000;
}
mp_falso = null;
mp_falso = new MediaPlayer();
String direttorio_wav, percorso_wav;
//-WAV--------------------------------------------------------------------------------------------------
try {
direttorio_wav = GLOBALE.direttorio_SDCARD + GLOBALE.direttorio_file;
percorso_wav = direttorio_wav + "beep_up.mp3";
mp_falso.setDataSource(percorso_wav);
mp_falso.prepare();
mp_falso.start();
mp_falso.pause();
}
catch (Exception e)
{
System.out.println (">>>>>>>>>>>>>>Errore in playSound_x " ); //in caso di eccezione ...
// textView01.setText("errore mp3 pause");
}
}
@Override
public boolean onCreateOptionsMenu(Menu menu) {
// Inflate the menu; this adds items to the action bar if it is present.
getMenuInflater().inflate(R.menu.main, menu);
return true;
}
@Override
public boolean onOptionsItemSelected(MenuItem item) {
// Handle presses on the action bar items
int i;
switch (item.getItemId()) {
case R.id.item1:
//openSearch();
for (i=0; i< GLOBALE.numero_materie; i++) {
GLOBALE.vettore_elenco_materie_ok_play[i] = false;
}
// GLOBALE.vettore_elenco_materie_ok_play[GLOBALE.numero_materie-1] = true;
return true;
// openSettings();
case R.id.item3:
//openSearch();
for (i=0; i< GLOBALE.numero_materie; i++) {
GLOBALE.vettore_elenco_materie_ok_play[i] = true;
}
return true;
// openSettings();
case R.id.item2:
//openSearch();
getWindow().addFlags(WindowManager.LayoutParams.FLAG_KEEP_SCREEN_ON);
return true;
// openSettings();
case R.id.item4:
//openSearch();
AlertDialog.Builder miaAlert = new AlertDialog.Builder(this);
miaAlert.setTitle("Info");
miaAlert.setMessage("Question to save " + num_risposte_al_salvataggio + "\n Question to exit " + (intervallo_spegnimento - conteggio_allo_spegnimento));
AlertDialog alert = miaAlert.create();
alert.show();
return true;
// openSettings();
case R.id.item5:
//openSearch();
esegui_il_timer_task = 0;
return true;
// openSettings();
case R.id.item6:
//openSearch();
esegui_il_timer_task = 1;
myTask = null;
MyTimerTask myTask2 = new MyTimerTask();
Timer timer2 = new Timer();
timer2.schedule(myTask2, 1000);
return true;
// openSettings();
case R.id.item7:
//openSearch();
esegui_il_timer_task = 2;
myTask = null;
MyTimerTask myTask1 = new MyTimerTask();
Timer timer1 = new Timer();
timer1.schedule(myTask1, 1000);
return true;
default:
return super.onOptionsItemSelected(item);
}
}
TIMER DI ESECUZIONE DEI FILE AUDIO
Definizione della classe timertask. Creazione dei timer da rispettare per mandare in play i file audio// Autore
// Ing. Paolo Sordi, via casilina n. 88 San Cesareo (Roma)
//
class MyTimerTask extends TimerTask {
public boolean materia_da_mandare_in_play = false;
public void run() {
if(timer != null) {
timer.cancel();
timer.purge();
timer = null;
}
if (esegui_il_timer_task == 0) return;
if (esegui_il_timer_task == 2) esegui_il_timer_task = 0;
Random r = new Random();
int i1;
System.out.println ("-------TimerTask-------S " );
if (GLOBALE.domanda_risposta == 1){
boolean non_trovato = true;
num_risposte_date++;
num_risposte_al_salvataggio--;
conteggio_allo_spegnimento++;
// textView01.setText("." + conteggio_allo_spegnimento);
/*
vettore = new short [4];
vettore [0] = GLOBALE.vettore_estrazione[indice];
vettore [1] = GLOBALE.vettore_scalata[indice];
vettore [2] = 1;
vettore [3] = 1;
GLOBALE.scrivi_file_informazioni(GLOBALE.vettore_nome_domande[indice], vettore);
*/
if (num_risposte_date % intervallo_salvataggio == 0) {
GLOBALE.salvataggio_automatico();
num_risposte_al_salvataggio = intervallo_salvataggio;
playSound_x ("beep_save.mp3");
}
if (conteggio_allo_spegnimento > intervallo_spegnimento) {
salva_e_chiudi();
}
while (non_trovato){
if (indice >-1) {
// GLOBALE.scrivi_file_informazioni(GLOBALE.vettore_nome_domande[indice], indice);
}
i1 = r.nextInt(GLOBALE.numero_elementi-1) + 1;
indice = GLOBALE.vettore_domande[i1];
// GLOBALE.leggi_file_informazioni(indice);
// System.out.println ("-controlla_se_la_materia_puo_andare_in_play- " + indice);
if (controlla_se_la_materia_puo_andare_in_play())
{
GLOBALE.vettore_scalata[indice]++;
materia_da_mandare_in_play = true;
}
else
{
materia_da_mandare_in_play = false;
}
// System.out.println ("-controlla_se_la_materia_puo_andare_in_play- ");
// System.out.println ("-------TimerTask-------Z " + i1 );
if (GLOBALE.vettore_scalata[indice] >= GLOBALE.vettore_estrazione[indice] && materia_da_mandare_in_play) {
non_trovato = false;
}
}
}
if (GLOBALE.domanda_risposta == 1 && controlla_se_la_materia_puo_andare_in_play())
{
playSound();
}
else
{
if (GLOBALE.domanda_risposta == 0 ) playSound();
}
Player_mp3.this.runOnUiThread(new Runnable() {
@Override
public void run() {
text_percorso.setText(stringa7 + nomefile );
// text_num_domande.setText(stringa4 + GLOBALE.numero_elementi + " - " + debug_ritardo + " - " + durata_wav);
text_num_domande.setText(stringa4 + indice);
textView7.setText("Topic");
if (aggiorna_edit_materia) {
editText1.setText(GLOBALE.vettore_materia[indice]);
}
aggiorna_edit_materia = false;
if (indice>=0 && GLOBALE.domanda_risposta == 0) {
editText1.setText(GLOBALE.vettore_materia[indice]);
}
calcola_percentuale (false);
aggiorna_durata();
}
});
if (GLOBALE.chiudi_applicazione) {
GLOBALE.salvataggio_automatico();
if (powerLock != null && powerLock.isHeld()) powerLock.release();
finish();
System.exit(0);
}
if (GLOBALE.domanda_risposta == 1) {
// tempo tra la domanda e la risposta
myTask = null;
MyTimerTask myTask = new MyTimerTask();
Timer timer = new Timer();
debug_ritardo = intervallo + durata_wav + GLOBALE.vettore_ritardo_risposta[indice];
timer.schedule(myTask, 1000*(intervallo + durata_wav + GLOBALE.vettore_ritardo_risposta[indice]));
}
// tempo tra la fine di una risposta e una nuova domanda
if (GLOBALE.domanda_risposta == 0) {
myTask = null;
MyTimerTask myTask = new MyTimerTask();
Timer timer = new Timer();
debug_ritardo = intervallo + durata_wav ;
timer.schedule(myTask, 1000*(intervallo + durata_wav));
}
}
}
public void salva_e_chiudi() {
GLOBALE.salvataggio_automatico();
if (powerLock != null && powerLock.isHeld()) powerLock.release();
finish();
System.exit(0);
}
PROGETTO DEGLI EVENTI ALL’INTERNO DELL’ACTIVITY
Gestione degli eventi di click sui bottoni del layout// Autore
// Ing. Paolo Sordi, via casilina n. 88 San Cesareo (Roma)
//
public void onClick(View v) {
// TODO Auto-generated method stub
if ( v.getId() == R.id.button8) {
Intent form_intent2 = new Intent(getApplicationContext(), Menu_principale.class);
startActivity(form_intent2);
}
if ( v.getId() == R.id.button1) {
GLOBALE.domanda_risposta = -1000;
myTask = null;
if(timer != null) {
timer.cancel();
timer.purge();
timer = null;
}
System.out.println ("STOP STOP STOP " ); //in caso di eccezione ...
if (mp != null) {
if (mp.isPlaying()) {
mp.stop();
}
}
button1.setEnabled(false);
button2.setEnabled(true);
}
if ( v.getId() == R.id.button2) {
GLOBALE.domanda_risposta = 1;
System.out.println ("START START START " );
myTask = null;
if(timer != null) {
timer.cancel();
timer.purge();
timer = null;
}
MyTimerTask myTask = new MyTimerTask();
Timer timer = new Timer();
timer.schedule(myTask, 1000*2);
button2.setEnabled(false);
button1.setEnabled(true);
}
if ( v.getId() == R.id.button3) {
GLOBALE.vettore_estrazione[indice]++;
calcola_percentuale (true);
}
if ( v.getId() == R.id.button4) {
if (GLOBALE.vettore_estrazione[indice] > 1) {
GLOBALE.vettore_estrazione[indice]--;
calcola_percentuale (true);
}
}
/* if ( v.getId() == R.id.button6) {
GLOBALE.vettore_ritardo_risposta[indice] += 1;
aggiorna_durata ();
}
if ( v.getId() == R.id.button5) {
if (GLOBALE.vettore_ritardo_risposta[indice] > 1) {
GLOBALE.vettore_ritardo_risposta[indice] -=1 ;
aggiorna_durata ();
}
}
*/
if ( v.getId() == R.id.button9) {
textView7.setText("Topic new");
GLOBALE.vettore_materia[indice] = editText1.getText().toString();
inserisci_la_nuova_materia_se_non_esiste ();
}
if ( v.getId() == R.id.button7) {
/*
mp.pause();
length=mp.getCurrentPosition();
if (GLOBALE.domanda_risposta == 1) GLOBALE.domanda_risposta = -1001;
if (GLOBALE.domanda_risposta == 0) GLOBALE.domanda_risposta = -1000;
*/
scelta_materia();
editText1.setText(GLOBALE.vettore_materia[indice]);
}
if ( v.getId() == R.id.button10) {
/*
mp.pause();
length=mp.getCurrentPosition();
if (GLOBALE.domanda_risposta == 1) GLOBALE.domanda_risposta = -1001;
if (GLOBALE.domanda_risposta == 0) GLOBALE.domanda_risposta = -1000;
*/
showMultipleChoiceItems("Topics");
}
}
CONNESSIONE CON L’AURICOLARE BLUETOOTH
Questa parte del software è stata realizzata quasi subito dopo il framework principale. L’obiettivo del programma è infatti quello di poter studiare anche quando manualmente si è impegnati in altre attività L’applicazione può essere controllata dai pulsanti di un auricolare bluetooth. Una volta stabilita la connessione la pressione dei pulsanti è un evento che corrisponde alla digitazione di un tasto della tastier (evento keydown)// Autore
// Ing. Paolo Sordi, via casilina n. 88 San Cesareo (Roma)
//
//The BroadcastReceiver that listens for bluetooth broadcasts
private final BroadcastReceiver mReceiver = new BroadcastReceiver() {
@Override
public void onReceive(Context context, Intent intent) {
String action = intent.getAction();
// Toast.makeText(BlueDetectSrv.this, "BT change received !", Toast.LENGTH_LONG).show();
if (BluetoothDevice.ACTION_FOUND.equals(action)) {
// Toast.makeText(BlueDetectSrv.this, device.getName() + " Device found", Toast.LENGTH_LONG).show();
editText1.setText("1");
}
else if (BluetoothDevice.ACTION_ACL_CONNECTED.equals(action)) {
editText1.setText("2");
}
else if (BluetoothDevice.ACTION_ACL_DISCONNECT_REQUESTED.equals(action)) {
editText1.setText("3");
}
else if (BluetoothDevice.ACTION_ACL_DISCONNECTED.equals(action)) {
editText1.setText("4");
salva_e_chiudi();
}
}
};
// --------------------------------------------------------
public boolean onKeyDown(int keyCode, KeyEvent event) {
// stringa4.setText("-" + keyCode);
if (keyCode == 127 || keyCode == 84) {
GLOBALE.vettore_estrazione[indice]++;
calcola_percentuale (true);
playSound_x ("beep_up.mp3");
}
if (keyCode == 88) {
GLOBALE.vettore_estrazione[indice]=1;
calcola_percentuale (true);
playSound_x ("beep_uno.mp3");
}
if (keyCode == 87) {
GLOBALE.vettore_materia[indice]= "xbug";
editText1.setText(GLOBALE.vettore_materia[indice]);
playSound_x ("beep_bug.mp3");
}
return super.onKeyDown(keyCode, event);
}
// Autore
// Ing. Paolo Sordi, via casilina n. 88 San Cesareo (Roma)
//
public void playSound (){
String percorso_wav;
String direttorio_wav;
//-WAV--------------------------------------------------------------------------------------------------
GLOBALE.vettore_scalata[indice] = 0;
System.out.println ("Playsound" ); //in caso di eccezione ...
direttorio_wav = GLOBALE.direttorio_SDCARD + GLOBALE.direttorio_file;
nomefile = GLOBALE.vettore_nome_risposte[indice];
GLOBALE.scrivi_stringa_file_cronologia("nomefile: " + nomefile);
if (GLOBALE.vettore_ritardo_risposta[indice] == -45645)
{
percorso_wav = direttorio_wav + nomefile;
try {
mp = new MediaPlayer();
mp.setWakeMode(getApplicationContext(), PowerManager.PARTIAL_WAKE_LOCK);
mp.setDataSource(percorso_wav);
mp.prepare();
durata_wav = (long)mp.getDuration();
durata_wav = durata_wav /1000;
mp.release();
mp = null;
// passiamo il file che deve essere in res
// creiamo il player passandogli l'inputstream e il tipo di file
GLOBALE.vettore_ritardo_risposta[indice] = (short)durata_wav;
}
catch (Exception e)
{
text_percorso.setText(stringa7 + nomefile + " error1 ...");
GLOBALE.vettore_ritardo_risposta[indice] = 1;
System.out.println ("sfore in playSound " ); //in caso di eccezione ...
GLOBALE.scrivi_stringa_file_cronologia("E1 nomefile: " + nomefile);
// text_percorso.setText(stringa7 + nomefile + " - Error");
}
}
if (GLOBALE.domanda_risposta == 1) {
direttorio_wav = (GLOBALE.direttorio_SDCARD + GLOBALE.direttorio_file);
nomefile = GLOBALE.vettore_nome_domande[indice];
}
GLOBALE.domanda_risposta += 1;
if (GLOBALE.domanda_risposta > 1) {
GLOBALE.domanda_risposta = 0;
}
percorso_wav = direttorio_wav + nomefile;
try {
mp = new MediaPlayer();
mp.setDataSource(percorso_wav);
// mp.setWakeMode(getApplicationContext(), PowerManager.PARTIAL_WAKE_LOCK);
mp.prepare();
durata_wav = (long)mp.getDuration();
durata_wav = durata_wav /1000;
mp.start();
// Toast.makeText(getBaseContext(), "Playing", Toast.LENGTH_SHORT).show();
mp.setOnCompletionListener(new OnCompletionListener() {
@Override
public void onCompletion(MediaPlayer mp) {
mp.release();
mp = null;
}
});
//text_percorso.setText(stringa7 + nomefile + " [" + durata_wav + " s]");
//passiamo il file che deve essere in res
//creiamo il player passandogli l'inputstream e il tipo di file
}
catch (Exception e)
{
text_percorso.setText(stringa7 + nomefile + " error2 ...");
System.out.println ("Errore in playSound " ); //in caso di eccezione ...
GLOBALE.scrivi_stringa_file_cronologia("E2 nomefile: " + nomefile);
}
}